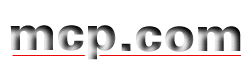
Day 7
Extending Your Web
Page Through Client-Side Script
Most of the focus for the week has been on the client. You have learned the basic
building blocks of building a better client Web page. On this last day of the first
week, you will learn a final piece to the client side of the application puzzle.
Client-side scripting can provide a dynamic experience for the user if used
in the right manner.
The first part of the day is an overview of how to successfully use scripting
code on the client. You will gain an understanding of the power and pitfalls of executing
script within the context of the user's browser. The lesson then defines VBScript
and JavaScript. These two scripting languages are the most popular and widely used
languages for coding client-side script. You also will learn how script code interacts
and coexists with your HTML code.
The latter part of the day provides you with some VBScript basics. This section
is not meant to be an exhaustive discourse on the topic of VBScript; however, it
will give you a pretty detailed overview on the features and capabilities of the
language. The last section teaches you how to use VBScript in your applications for
the Web. In this section, specific examples of VBScript and web page interaction
are covered.
Scripting for Success
HTML provides a standard method for Web browsers to render web pages but lacks
the power to interact with the user. Scripting languages were created to provide
a method for the user to interact with the web page. The user metaphor for the Web
has gone from information gathering to true interaction. Web-based applications must
have a way to interact with the user. HTML does a fairly good job of formatting documents
for the Web but can't handle application processing.
The first applications for the Web used the server to execute special instructions
and application logic. Program interfaces on the server like CGI have provided this
processing in the past. This model is very inefficient in that the information and
logic that could be verified and processed on the client is constantly passed back
and forth between the client and the server. This situation creates more traffic
across the network and additional processing for the server machine.
Client-side script can help alleviate this inefficient process. With the advent
of scripting languages, the Web browser can process certain functions on the client
through the use of script code embedded in the HTML. Script is normally used for
user-initiated events like form activities or mouse events. For example, you can
use client-side script to verify that a user entered the right type of information
into a field on a form. A user that clicks the mouse or moves the mouse over a certain
area of the web page could also trigger the execution of client-side script.
NOTE: All references to script in this
chapter pertain to the implementation of script within an HTML web page on a client
machine unless noted otherwise. Day 11, "Extending Your Application Through
Active Server Script," covers the concepts of implementing script on the server.
The advantages of using client-side script in your HTML web page include
- Increased interaction with the user
- Shared processing of simple tasks with the server
- Integration of multiple objects like ActiveX controls and HTML form controls
- More responsive web pages
There are some limitations to executing script on your client. First, security
models are still being developed for the different scripting languages. Some people
might argue that there is no definitive model. Most scripting languages were specifically
designed with limitations that prevent the code from performing destructive actions
on the client machine like destroying files. For instance, scripting languages cannot
perform file I/O.
Another limitation to scripting languages is the lack of support for defining
different data types. For example, VBScript only supports one data type. If you want
to use a different data type, you have to change the type programmatically. The key
point is that you must be careful in coding your client-side script. Both Microsoft
and Netscape have attempted to implement security measures for their languages. You
should, however, be cognizant of the fact that this code is still executing on an
unsecured client machine.
You should definitely implement client-side script as a part of your Web-based
application. The benefits far outweigh the limitations as long as you construct your
code in the right manner. In this respect, a Web-based application is analogous to
a movie. A movie must contain a good script no matter how good the actors are. Likewise,
your applications must contain effective script to produce the desired results for
your applications. You will learn how to implement effective script later in the
day.
The Marriage of HTML and Scripting
Languages
Scripting code is embedded within the confines of an HTML page. The <SCRIPT>
and </SCRIPT> tags separate the script from the rest of the HTML.
Listing 7.1 demonstrates the structure of an HTML document that contains scripting
code.
Listing 7.1. VBScript code example.
<HTML>
<HEAD>
<TITLE>VBScript Page </TITLE>
</HEAD>
<BODY>
<P>HTML Paragraph Text
<SCRIPT LANGUAGE = "VBScript">
<!--
...VB Scripting Code is here
!-->
</SCRIPT>
</BODY>
</HTML>
In this example, the HTML document uses VBScript as its language. You can see
the type of scripting language in the following line:
<SCRIPT LANGUAGE = "VBScript">.
The next line contains a comment tag that denotes the beginning of the scripting
code. A comment tag is used for those browsers that can't execute script. Scripting
code is hidden from these browsers and treated as if the code were comments. The
next line contains the actual script. Your scripting code extends for multiple lines
within your document. A closing comment tag is placed at the end of the script. This
tag is followed by an ending </SCRIPT> tag.
NOTE: Script can be displayed in the <HEAD>
and <BODY> sections. If the script is included in the <HEAD>
section, the code is interpreted before the page is fully downloaded.
Scripting languages like VBScript and JavaScript are interpreted languages. An
interpreted language must be translated by another program at runtime to be able
to execute. This interpreter program performs the same duties as a person who acts
as a translator between people who speak different languages. The interpreter listens
to the speech of one person and translates those words into words that the second
person can understand. In a similar fashion, an interpreter program must translate
the language of a scripting program to a language that the client machine can understand.
Given the code in Listing 7.1, the VBScript interpreter looks for the <SCRIPT>
tags and processes all of the code in between.
For browsers that can read and support client script, the integration and marriage
of HTML and script can be quite harmonious. You will definitely see the benefit of
using scripting code on the client machine when you're building an application for
the Web. Client-side script can significantly enhance the use of objects like Java
applets, ActiveX controls, and HTML form controls.
You're probably wondering about the different scripting languages that are available.
The next two sections provide a definition and overview of the two most widely used
scripting languages.
What Is VBScript?
VBScript is a subset of the Visual Basic language and is Microsoft's entry into
the Internet scripting languages arena. For developers who are familiar with Visual
Basic, you will recognize much of the VBScript language and syntax. VBScript is very
easy to learn and implement. Microsoft has created and optimized this scripting language
specifically for the Internet. Microsoft's Internet Explorer 3.0 supports the use
of VBScript by providing the VBScript run-time interpreter.
VBScript uses procedures and functions to process your application needs.
What Is JavaScript?
JavaScript performs the same type of scripting extensions as VBScript. Netscape
collaborated with Sun Microsystems to develop JavaScript as a scripting language
to accentuate the Java programming language. Like VBScript, JavaScript is interpreted
at runtime. You must use a browser that includes a JavaScript runtime interpreter.
Many publications use the terms JavaScript and Java interchangeably. JavaScript
is not Java. The Java programming language enables you to create applets and applications.
These programs are precompiled programs that execute specific functions. You can
insert Java applets into your web page. You also can call Java programs on the server
to process more extensive application logic.
JavaScript, on the other hand, is an interpreted scripting language that resides
within the context of an HTML page. The browser, with the help of a JavaScript run-time
interpreter, translates the script along with the rest of the HTML when the web page
is downloaded from the server. JavaScript, by nature, doesn't possess the strength
or robustness of the Java programming language. JavaScript borrows much of its syntax
from the Java language. Listing 7.2 demonstrates the format for JavaScript code within
an HTML document.
Listing 7.2. JavaScript code example.
<HTML>
<HEAD>
<TITLE>JavaScript Page </TITLE>
</HEAD>
<BODY>
<P>HTML Paragraph Text
<SCRIPT LANGUAGE = "JavaScript">
<!--
...JavaScript Scripting Code is here
// -->
</SCRIPT>
</BODY>
</HTML>
In Listing 7.2, notice the closing comment tag is different than the closing comment
in the previous VBScript example. For JavaScript, a closing comment tag is denoted
by two forward slashes. Also, the <SCRIPT LANGUAGE> tag set to "JavaScript"
indicates that the scripting language is JavaScript.
VBScript and JavaScript are similar in coding structure. JavaScript, like VBScript,
doesn't support specific type casting of variables. An integer is represented in
the same way as a string. Also, JavaScript makes use of functions, methods, and properties,
similar to VBScript, to accomplish its tasks. Functions are defined in the following
section, "VBScript Basics." Methods and properties are defined on Day 13,
"Interacting with Objects and ActiveX Controls."
NOTE: At the time of the writing of this
book, VBScript and JScript were only supported by Internet Explorer 3.0 and higher,
while JavaScript was supported by both Internet Explorer and Netscape Navigator and
Communicator.
Listing 7.3 shows a sample page implemented with VBScript.
Listing 7.3. Hello world with VBScript.
<HTML>
<HEAD>
<SCRIPT LANGUAGE="VBScript">
<!--
Sub DisplayHello_onClick()
MsgBox "Hello world!", 0, "VBScript"
end sub
-->
</SCRIPT>
<META NAME="GENERATOR" Content="Microsoft Developer Studio">
<META HTTP-EQUIV="Content-Type" content="text/html; charset=ISO-8859-1">
<TITLE>Sample VBScript Page</TITLE>
<H1>This is VBScript </H1>
</HEAD>
<BODY>
<CENTER>
<FORM>
<INPUT TYPE=BUTTON VALUE="Display VBScript" NAME="DisplayHello">
</FORM>
<BR><BR><BR>
</CENTER>
<a href="HelloWorld.htm">Hello World Home Page</a>
</BODY>
</HTML>
Listing 7.4 shows the same sample page implemented with JScript.
Listing 7.4. Hello world with JavaScript.
<HTML>
<HEAD>
<SCRIPT LANGUAGE="JScript">
<!--
function DisplayHello() {
alert("Hello world!");
}
//-->
</SCRIPT>
<META NAME="GENERATOR" Content="Microsoft Developer Studio">
<META HTTP-EQUIV="Content-Type" content="text/html; charset=ISO-8859-1">
<TITLE>Sample JavaScript Page</TITLE>
<H1>This is JavaScript</H1>
</HEAD>
<BODY>
<CENTER>
<FORM>
<INPUT TYPE=BUTTON VALUE="Display JavaScript" onclick="DisplayHello()">
</FORM>
<BR><BR><BR>
</CENTER>
<a href="HelloWorld.htm">Hello World Home Page</a>
</BODY>
</HTML>
These two code examples show some differences between VBScript and JavaScript.
The first difference is the format of a JavaScript function. The syntax is very similar
to the C++ language, which uses braces to organize a block of code statements and
semicolons to signify the end of a statement.
Another difference is the method that is used to call a JavaScript function. Notice
in the JavaScript example that the word onclick is used to call the function.
In the VBScript code, the word NAME is used to activate the procedure. If
you aren't familiar with C++ or Java, VBScript may seem like the more intuitive language.
Both languages support your needs for providing robust, client-side functionality.
Microsoft has reverse engineered the JavaScript code into its own implementation
called JScript. Both implementations are pretty much the same, although peculiarities
do exist. Microsoft and Netscape agreed in November 1995 to define a single specification
for JavaScript that will be managed by the European Computer Manufacturers Association
(ECMA) standards body. This single specification hopefully avoids the problem that
people have experienced with other "open" technologies, such as the UNIX
operating system.
NOTE: Visual InterDev natively supports
the use of JScript with its editors and tools. When you choose a scripting language
for your projects, you must either choose VBScript or JScript. You can, however,
create a web page containing JavaScript with another editor, such as Notepad, and
insert this file into your project.
VBScript Basics
This part of the lesson teaches you the basic building blocks for creating VBScript
code. In the section "Using VBScript to Extend Your Web Page," you apply
these lessons and discover how to integrate VBScript into a web page.
Understanding Procedures
VBScript uses procedures to provide a home for its code. You're probably familiar
with the concept of using procedures. Most programming environments, regardless of
the language, use procedures as their basic foundation. Procedures provide a logical
container for groups of related code.
New Term: A procedure is a logical
grouping of code statements that works together to complete a specific task. Procedures
can be called from within your application and also can call other procedures.
VBScript contains three types of procedures:
- 1. Sub procedures
2 Functions
3.Event procedures
Sub Procedures
A sub procedure is a group of related VBScript code statements that complete a
task but do not return a value to the calling program. I stated before that a procedure
is called from your application or another procedure. When a program or procedure
calls a sub procedure, the caller asks the procedure to perform a task. The calling
program isn't interested in receiving anything in return. This process is analogous
to a person calling a restaurant for carry-out food. The person calls the restaurant
to prepare the food, and the person then drives to the restaurant to pick up the
food. I will contrast this process with that of a function in the next section, so
keep the food analogy fresh on your mind.
When a sub procedure is invoked, program control is temporarily passed to the
called procedure. A sub procedure is denoted by the Sub and End Sub
keywords. You can think of these keywords as tags that signify the beginning and
ending of the procedure. They are similar in nature to HTML tags that mark the beginning
and ending of an HTML element. The following code segment illustrates the basic structure
of a sub procedure:
Sub CalculateTotal (A,B)
Total=A*B
MsgBox "The total is " & Total
End Sub
In this example, CalculateTotal is the name of the sub procedure. A
and B refer to arguments that are passed by the calling program. These arguments
are optional. You can pass up to n arguments to the called sub procedure. You also
may develop procedures that don't need parameters to be passed. For those sub procedures,
the parentheses are optional.
New Term: An argument is a variable
that a procedure needs to complete its task. You can specify a number of arguments
to be passed as long as they're in the correct order.
You can pass arguments by value to the procedure. You specify an argument to be
passed by value by placing ByVal in front of the argument.
New Term: By value means that a copy
of the variable's value is passed to the procedure. The procedure can use this value
as well as make changes to it within the scope of the procedure. Because the variable
is passed as a copy, changes that are made by the procedure to the value of the variable
don't affect the original variable.
The following code segment demonstrates how to pass a variable by value:
Sub CalculateTotal(ByVal A, ByVal B).
Arguments also can be passed by reference. This method is the default method of
passing a variable. This method differs from the traditional way that other development
tools such as Visual Basic construe passing a variable by reference.
New Term: By reference in VBScript
means that a variable's value is passed to the procedure as read-only. The procedure
can read the value but can't make any changes to it.
In Visual Basic and other tools, passing a variable by reference enables you to
access the storage of the original variable and changes the contents of the original
variable. After the procedure has completed, the variable reflects the changes when
the calling program tries to access its contents. VBScript doesn't enable you to
alter the contents of the original variable.
You do not have to explicitly state that you're passing a variable by reference.
The following code example shows how to pass a variable by reference:
Sub CalculateTotal(A, B).
You should develop descriptive names for your sub procedures so that anyone who
uses the procedure will know what it does. I prefer to name my procedures using a
verb-object nomenclature. For example, if I developed a procedure to format a date
to display to the user, I would name that procedure FormatDate. Format
is the verb that tells what the procedure is doing, and Date represents
the object that is the object of the action. The preceding example multiplied two
numbers to calculate a total, hence the name CalculateTotal. Valid characters
to include in your procedure name include letters and numbers as long as the first
character is not a number. You can't use symbols in your procedure name. VBScript
performs error checking on your names to validate their syntax.
To call a sub procedure, you simply enter the name of the procedure. You also
can use the optional Call keyword to activate a procedure. The following
examples demonstrate how to call a sub procedure. The first example uses the Call
keyword while the second example only states the name of the procedure. To call the
CalculateTotal sub procedure, you can use
Call CalculateTotal(A, B)
or
CalculateTotal(A, B)
If the procedure that you're calling requires arguments, place the arguments within
optional parentheses after the name of the procedure. To call the FormatDate
procedure that doesn't require parameters, enter
FormatDate()
or
FormatDate
VBScript provides you with a lot of flexibility when calling a sub procedure.
You may want to explicitly call procedures with the Call keyword so that
you can distinguish the difference between a sub procedure and other elements within
your code, such as variables.
Functions
The second type of procedure is a function. Similar to a procedure, a function
is a collection of VBScript statements that work together to perform a task. The
difference between a procedure and a function is that a function can return a value.
New Term: A function is a group of
code statements that collaborate to accomplish a task. A function is similar to a
procedure in that a function can accept arguments and be called from the application
and other procedures. A function can return a value to the calling program.
A function is denoted by the Function and End Function keywords.
The following code example demonstrates the structure of a function.
Function Function Name(Argument 1, Argument 2,..., Argument n)
...Function Code
End Function
The structure of a function is very similar to the structure of a sub procedure.
The same rules concerning sub procedure names and arguments apply to functions. I
stated that the distinguishing factor concerning a function was the ability to return
a value to the calling function.
When I explained the concept of a sub procedure, I used the analogy of calling
a restaurant to order carry out. You call the restaurant, order the food, and pick
it up from the restaurant. Extending the analogy, the function represents a person
who calls and orders food to be delivered. You call the restaurant and ask for a
mushroom pizza to be delivered to your house. The restaurant informs you that they
need to cook the pizza, and it will be delivered in 30 minutes. The delivery person
drives to your house and delivers the pizza. You can then use the pizza to feed yourself
and your family. Similarly, a program makes a request of a function expecting to
get something in return. When the function finishes executing its code, it sends
a value back to the calling program. Listing 7.5 illustrates how a procedure can
call a sample VBScript function and receive a value in return.
Listing 7.5. Returning a value.
<SCRIPT LANGUAGE="VBScript">
<!--
Sub ConvertTemp()
temp = InputBox("Please enter the temperature in degrees Fahrenheit:", 1)
MsgBox "The temperature is " & Celsius(temp) & " degrees C."
End Sub
Function Celsius(fDegrees)
Celsius = (fDegrees - 32) * 5 / 9
End Function
!-->
</SCRIPT>
In this code example, the sub procedure prompts the user to enter a temperature
in degrees Fahrenheit. After the user enters the number, the function is called to
convert the temperature to Celsius. Notice that the function variable, Celsius,
which captures the converted temperature, is the same as the name of the function.
In order to return a value back to the calling program, you must specify a variable
to have the same name as the function. By design, the function returns a value through
the use of its own name as the variable.
The function in Listing 7.5 contained only one line of code. Most of your functions
will contain multiple lines of VBScript code. For this reason, you should make it
a practice to populate the function variable in the last line of the function code.
Listing 7.6 shows a sample function that contains multiple lines of code.
Listing 7.6. Formatting the function
variable on the last line of code.
<SCRIPT LANGUAGE="VBScript">
<!--
Function CalculateAverage(A,B)
Dim Total
Total = (A*B)/2
CalculateAverage = Total
End Function
!-->
</SCRIPT>
In this example, a temporary variable, Total, is established to hold
the value of the calculated average. On the last line of the function, CalculateAverage
is set to the value that is stored within the Total variable. Although a
simple example, I think you can extrapolate the significance if your function code
has many lines. Using this standard can provide meaning to your functions. You will
always know to look at the last line of the code for the value that is being returned
to the calling program. Assigning the function variable on the last line of the function
also prevents this statement from getting lost in the shuffle of your code.
Calling a function is different from calling a procedure. Because a function returns
a value, you must be prepared to do something with the value when it returns from
the function. The syntax for calling a function is
Return_Variable = Function Name(Argument 1, Argument 2,..., Argument n)
where Return_Variable is the name of the variable that will store the value that
is returned from the function. To call the CalculateAverage function, you
could enter either
Average = CalculateAverage(A,B)
or
Average = CalculateAverage A,B
Notice that the parentheses are optional. For a function that doesn't require
arguments, you can enter either
Date1 = FormatDate()
or
Date1 = FormatDate
Notice again that the parentheses are optional.
Functions are very useful when you need to complete a task and then return the
results back to the calling program.
Event Procedures
The first two procedures that you have learned about are procedures that you can
create. The event procedure is different from sub procedures and functions in that
it is constructed automatically for you by the objects and controls that you use
to build your application. Event procedures also differ from sub procedures and functions
in the manner that they are initiated. The browser calls event procedures automatically,
based on user actions and requests. With sub procedures and functions, you must call
them within the context of your program.
New Term: An event procedure is a group
of code statements activated by a user-initiated event. This action could be the
result of a user action such as clicking a button. An event procedure also could
be triggered by a system action where the user has made a request of the system and
the system needs to respond.
When you use objects such as ActiveX controls to create your application interface,
standard events are associated with these controls. For example, a user can click
a button; therefore, a standard event for a button is the On_Click event.
This event is triggered and its code is executed any time the user clicks the button.
The structure for your event procedure code is constructed automatically based on
the controls that you select. You must fill in the blank with any code that you want
processed when the event is initiated.
NOTE: On Day 4, "Creating Your First
Visual InterDev Project," you received a glimpse of event procedures when you
inserted some code for the Say Hello button. You used the Script Wizard to generate
some of the code for you. You will learn more about using the Script Wizard to generate
your scripting code for control events on Day 15, "Integrating Objects into
Your Applications."
You don't have much choice in naming your event procedures. Most of the time this
name is generated for you. The standard naming convention for an event procedure
is as follows:
Sub ControlName_EventName()
ControlName is the name of the control and EventName refers to the name of the
event. For example, an event procedure for a click event associated with the Submit
button might be called
Sub cmdSubmit_OnClick
NOTE: The control name is based on the
ID property value that you establish when you place the control within your application.
In the preceding example, I placed a button on a web page and changed the value for
the ID property to cmdSubmit. Naming conventions for controls are explained
in more detail on Day 13.
Event procedures are preconstructed programming shells that a control provides
for you. Each control will have a different set of events associated with it. Event
procedures serve as a helpful reminder to think about the various user and system
actions that can occur within your application. Based on these actions, you can then
provide the logic process and handle the application requests.
Procedures and HTML
As you can see, procedures provide the foundation and residence for your VBScript
code. Although you can place script outside the confines of a procedure, most of
your application logic resides within a procedure. You should generally place your
VBScript code within the <HEAD> section of an HTML document for readability.
You may be tempted to separate your procedures into different script sections as
in Listing 7.7.
Listing 7.7. Separating the script.
<SCRIPT LANGUAGE="VBScript">
<!--
Function CalculateTotal(A,B)
Total = A+B
CalculateTotal = Total
End Function
!-->
</SCRIPT>
<SCRIPT LANGUAGE="VBScript">
<!--
Function CalculateAverage(A,B)
Dim GradeAverage
GradeAverage = (A*B)/2
CalculateAverage = GradeAverage
End Function
!-->
</SCRIPT>
While VBScript enables you to divide your script into separate sections, as in
Listing 7.7, it isn't a good habit to develop from a maintainability and readability
standpoint. You should instead make it a habit to place all of your code into one
script section. This practice enables you, as well as others who may use your code,
to easily understand and locate your scripting code.
Summarizing Procedures
Although this section on procedures may have been refresher for some of you, I
hope you have gained a better understanding of the basic building block for your
code. VBScript procedures are very similar to Visual Basic and other development
tools. Table 7.1 summarizes the three types of procedures and provides a description
of each type.
Table 7.1. VBScript procedures.
Procedure |
Description |
Sub Procedure |
Processes a group of related code statements |
Function |
Processes a group of related code statements and returns a value to the calling program |
Event Procedure |
Control-specific procedure that processes user or system events |
Understanding Variables
I have mentioned the term variable several times today. I am sure that you have
used variables to develop your applications, but I did want to define the term and
outline how you can use variables within the context of VBScript.
New Term: A variable serves as a placeholder
for some type of information.
You can use variables to store information that your application logic will need
at some point in time. You can access the value of the variable as well as modify
its contents.
Types of Variables
There is only one data type for a VBScript variable. The variant serves as the
data type for all of the variables that you create within your VBScript code. A variant
is very flexible in that it can hold almost any type of information.
New Term: A variant is a special data
type that can store all types of information, including strings, numbers, dates,
and objects such as ActiveX controls.
When you define a variable, you don't have to state an explicit data type. In
other programming languages, you have to specifically define a data type for the
variable such as an integer or a date. All variables in VBScript are defined as variants.
You don't have to worry about specifying a data type for your variables. When
you assign a value to a variable, VBScript stores additional subtype information
about the data that's being held in the variable. This subtype, or category, information
helps VBScript determine the usage of variants based on the variable's context. For
instance, if you want to add two number variables that are stored as variants, VBScript
assumes that the variables are numbers and treat them as such. Textual information
is handled in a similar manner, based on the internal subtype of the variable. Table
7.2 describes the different subtypes for a variant data type.
Table 7.2. Variant subtypes.
Subtype |
Description |
Boolean |
Contains a value of either True or False |
Byte |
Stores integers between 0 and 255 |
Integer |
Contains a number between -32,768 and 32,768 |
Long |
Contains an integer between -2,147,483,648 and 2,147,483,647 |
Single |
Contains single precision, floating point data with a range from -1.4E-45
to -3.4E38 for negative numbers and 1.4E-45 to 3.4E38
for positive numbers |
Double |
Contains double precision, floating point, or decimal data with a range from -4.9E-324
to -1.8E308 for negative numbers and 4.9E-324 to 1.8E308
for positive numbers |
Date/Time |
Contains a date including time information between January 1, 100, and December 31,
9999 |
Empty |
Contains 0 for numbers and "" for strings; represents a variable that hasn't
been assigned a value |
Error |
Contains a VBScript error number |
Null |
Variable that contains no data; represents a variable that has been assigned a value
of nothing |
String |
Contains alphanumeric information up to 2 million characters |
Object |
References an object like an ActiveX control |
Exposing the Variables Data Type
You can determine and change the subtype that VBScript selects for your variable.
There are two ways to discover the subtype. First, you can use the VarType
function. This function enables you to request the subtype of a variable. The syntax
for this function is as follows:
VarType(VariableName)
VariableName is the name of the variable that you are inquiring about. Listing
7.8 illustrates the use of the VarType function:
Listing 7.8. Determining the subtype
of a variant.
<SCRIPT LANGUAGE="VBScript">
<!--
Function DisplaySubtype(TestVariable)
Dim VariableSubtype
VariableSubtype = VarType(TestVariable) `
ÂDetermines the variable subtype
MsgBox "The subtype for the variable is " & VariableSubtype `
ÂDisplays the subtype
DisplaySubtype = VariableSubtype `Assigns the value of the subtype
Âto the function variable
End Function
!-->
</SCRIPT>
Listing 7.8 is included on the CD-ROM with this book. You can use this function
to determine the subtypes of variables within your application. This function requires
that a variable be passed as an argument. The function determines the subtype for
this variable and passes the value back to the calling program. I created it as a
function that displays a message box as well as returns the value of the variant
subtype.
The VarType.htm file on the CD-ROM contains the entire web page that
calls the function. To test the function, I populate the argument TestVariable
before it's passed to the function. When the web page is loaded, a message box displays
the value of the subtype. I used the message box for testing purposes only as well
as the hard-coding of the TestVariable. To use this function with your application
code, you should copy the function code and insert it into your <SCRIPT>
section. Also, if you want to use the return variable but not display the message
box, remove the MsgBox line of code.
The second method to determine the subtype of a variant is to use some special
functions provided with VBScript. These functions perform a check for a specific
data type. After the check is performed, the functions return a value of True
or False, indicating whether the variable matches the specific data type
of the function. The following list shows the type of VBScript functions that are
available:
- IsArray
- IsDat
- IsEmpt
- IsNul
- IsNumeri
- IsObjec
The syntax for each of these functions is as follows:
FunctionName(VariableName)
For example, to call the IsDate function, you would enter
IsDate(TestDate)
Remember that these functions return a value of either True or False.
For this reason, the most typical use of these functions is in the context of testing
whether the return value of the function is True or False. This
test can be performed using an If...Then...Else statement, which is covered
in a later section, "Controlling the Flow of the Program."
NOTE: Refer to Appendix D, "VBScript
Language Reference" to discover more about these functions as well as other
functions supplied by VBScript.
Changing the Variables Data Type
VBScript also supplies functions to alter the internal data type of a variable.
You can use these functions to specifically change the variable subtype that is assigned
by VBScript for the variant. Table 7.3 displays the available VBScript functions
to change a variant's subtype.
Table 7.3. VBScript subtype conversion functions.
Function Name |
Description |
CBool |
Converts subtype to Boolean |
CByte |
Converts subtype to Byte |
CDate |
Converts subtype to Date |
CDbl |
Converts subtype to Double |
CInt |
Converts subtype to Integer |
CLng |
Converts subtype to Long |
CSng |
Converts subtype to Single |
CStr |
Converts subtype to String |
The syntax for calling these functions is similar to the functions that determine
the subtype for a variant. For example, to call the CInt function, you would
enter
CInt(OriginalVariable)
This function converts the data to the integer format. For all of these functions,
you need to assign the return value to a variable:
ChangedVariable = CInt(OriginalVariable)
NOTE: Refer to the section entitled "Controlling
the Flow of the Program" for a detailed code example outlining the use of these
conversion functions.
Defining the Scope of a Variable
Variables can be used within the context of a procedure. You also can share variables
across all of your procedures. The scope of a variable determines its availability
to your code.
New Term: Scope refers to the ability
of a code statement to access a certain variable's contents. The scope of a variable
determines the context for usage by your application.
Variables consist of two types of scope--procedure-level and script-level. Procedure-level
scope, sometimes called local scope, refers to variables that are declared within
a procedure. Procedure-level variables can only be used and accessed within the context
of that procedure.
Script-level scope refers to variables that are defined outside your procedures.
Script-level variables can be recognized across all of your procedures. The lifetime
of your variable signifies the length of time that the variable exists. Lifetime
and scope are closely tied together. While scope determines what code statements
have access to the variable, lifetime indicates how long the variable exists in memory.
For procedure-level variables, the variable exists only for the life of the procedure.
When the procedure ends, the variable no longer exists. Script-level variables exist
until the script finishes processing.
You will constantly have a need to use variables. They are a powerful part of
any programming language. As you progress through the next few weeks, the proper
use of variables will become very evident.
Controlling the Flow of the Program
So far, you have learned about structuring your code through the use of procedures.
You also have recognized that variables are a necessary component of any set of code
statements. With all of this capability, how do you control your program's flow while
unleashing the potential of your code? VBScript, like other programming languages,
provides control structures that enable you to designate how your script is executed.
Control structures are analogous to highway signs that direct you to the right place.
You make decisions, based on these signs, about where to turn and which direction
to drive. Similarly, control structures help your code make decisions about which
logic to execute. This section covers the basic control structures that are available
within VBScript. This section serves as a refresher for those experienced Visual
Basic programmers.
If...Then...Else
This control structure is used to evaluate if a condition is True or
False and compares the values of variables. The following code example demonstrates
the use of this control structure:
If RoundWorld = True Then
MsgBox "Sail around the world!"
Else
MsgBox "Don't go! You'll fall off the earth!"
End If
Notice the structure of the If...Then...Else statement. You're basically
telling your program to evaluate if a condition is true. If it is, you want to execute
one piece of code. If the condition isn't true, you want to execute another piece
of code. There are several variations to this control structure. The first variation
involves only executing a piece of code if a situation is true. You don't care if
the situation is false. For this situation, you would enter the following:
If RoundWorld = True Then MsgBox "Sail around the world"
Another variation that is similar to the preceding example is if you want to run
multiple lines of code when a situation is true. For this scenario, you would enter
If RoundWorld = True Then
PackedBags = True
lblHouse.Caption = "Gone Sailing"
MsgBox "Sail around the world!"
End If
The End If statement is used in this example to signify the end of the
code. You must include this statement when executing multiple lines of code within
an If...Then statement.
You also can use this control structure to evaluate and compare the values of
variables. For this kind of comparison, you need to use the VBScript comparison operators.
NOTE: VBScript contains comparison, arithmetic,
and logical operators. Comparison operators, as the name states, enable you to compare
variables. Arithmetic operators enable you to perform mathematical operations between
numbers and variables. Logical operators assist you in testing the validity of one
or more variables For a comprehensive reference concerning comparison operators,
refer to Appendix D.
The following code example demonstrates the use of a comparison operator within
an If...Then...Else statement:
If Age >12 Then
MsgBox "You are a teenager."
Else
MsgBox "You are not a teenager."
End If
A final variation includes the ability to construct multiple tests. You can use
the ElseIf statement to construct another test within your If...Then...Else
statement. The most common use of this structure is when you have multiple comparisons
to perform. The following code example illustrates the use of this construct:
If Age <= 12 Then
MsgBox "You are a not a teenager."
ElseIf Age < 20 Then
MsgBox "You are a teenager."
ElseIf Age > 40 Then
MsgBox "It's all downhill from here."
End If
Notice from the previous example that you can construct multiple ElseIf
statements within an If...Then...Else statement.
Select Case Statements
Select Case statements are similar in function to the If...Then...Else
statement. Select Case statements enable you to execute code based on the
value of an expression. You will want to replace an If...Then...Else statement
with the Select Case statement when you have multiple conditions to test.
How many ElseIf statements are too much? I usually start to consider
a Select Case statement after the third test of a variable. In other words,
Listing 7.9 would be a good candidate for a Select Case statement.
Listing 7.9. Unwieldy If...Then...Else
statement.
<SCRIPT LANGUAGE="VBScript">
<!--
Sub DisplayName(Name)
If Name = "Bob" Then
MsgBox "Your name is Bob."
ElseIf Name = "Mike"
MsgBox "Your name is Mike."
ElseIf Name = "Gina"
MsgBox "Your name is Gina"
ElseIf Name = "Steve"
MsgBox "Your name is Steve"
End If
End Sub
!-->
</SCRIPT>
Listing 7.10 shows what the previous listing would look like as a Select Case
statement.
Listing 7.10. Providing structure
through a Select Case statement.
<SCRIPT LANGUAGE="VBScript">
<!--
Sub DisplayName(Name)
Select Case Name
Case "Bob"
MsgBox "Your name is Bob."
Case "Mike"
MsgBox "Your name is Mike."
Case "Gina"
MsgBox "Your name is Gina"
Case "Steve"
MsgBox "Your name is Steve"
Case Else
MsgBox "You don't have a name"
End Select
End Sub
!-->
</SCRIPT>
The first statement in the Select Case statement provides the expression
to be tested. This expression must have a distinct value. In other words, you can't
use a Select Case statement to make comparisons between variables. The lines
denoted by the Case statement signify the comparison value for each set
of code statements. VBScript traverses the list of Case statements, comparing
each value to the test expression. If it finds a match, the code for that Case
statement is executed. If no match is found, the code within the Case Else
statement is executed.
NOTE: It's a very good programming practice
to use the Case Else statement even if you think you have covered all of
the possible values in the Case statements. Your code will crash if you
don't provide a parachute for the script to execute in case of emergency.
I have provided a function included on the CD-ROM with this book that determines
the type of conversion that you want to perform and then converts the variable subtype.
This function demonstrates the use of a Case statement in combination with
some of the previous functions you learned about earlier today. Listing 7.11 displays
the code for this function.
Listing 7.11. Changing a variants
data type.
<SCRIPT LANGUAGE="VBScript">
<!--
Function ConvertSubtype(ConversionType,OriginalVariable)
Dim ChangedVariable
Select Case ConversionType
Case "Boolean"
ChangedVariable = CBool(OriginalVariable)
Case "Byte"
ChangedVariable = CByte(OriginalVariable)
Case "Date"
ChangedVariable = CDate(OriginalVariable)
Case "Double"
ChangedVariable = CDbl(OriginalVariable)
Case "Integer"
ChangedVariable = CInt(OriginalVariable)
Case "Long"
ChangedVariable = CLng(OriginalVariable)
Case "Single"
ChangedVariable = CStr(OriginalVariable)
Case "String"
ChangedVariable = CStr(OriginalVariable)
Case Else
ChangedVariable = ""
End Select
ConvertSubtype = ChangedVariable
End Function
!-->
</SCRIPT>
For...Next Loops
The For...Next loop is a widely used method to control the flow of your
code. You can use a For...Next loop to execute a group of code statements
a specified number of times. A counter is used to control the number of times that
the code is executed. By default, the counter is increased by one for each iteration
of the loop. You can set the starting value of the counter as well as the value of
the increment. You also can specify that the counter be decremented with each loop
iteration. The format of a For...Next loop is as follows:
For counter = beginning to end Step increment
Execute Code segment
Next
counter represents the variable that is going to be incremented, beginning signifies
the beginning number of the counter, end represents the ending value for the counter,
and increment specifies how much to increase or decrease the counter after each iteration
of the loop. The Step statement is optional.
Do Loops
The Do loop provides another popular way to execute your code multiple
times. The Do loop can be implemented in a variety of ways. You can use
the While keyword to execute a block of code as long as a condition is true.
The behavior of the loop changes based on where the While keyword is placed
in the loop. If the While keyword is placed in the opening line of the loop,
VBScript first checks to see if the condition is true before executing the code segment.
The syntax for the Do...While loop is
Do While condition
...block of code statements
Loop
You also can place the While keyword at the end of the loop to execute
the code at least once before exiting the loop. The syntax for the Do...Loop...While
is
Do
...block of code statements
Loop While condition
You also can use the Until keyword to execute a block of code until a
condition becomes true. The same rules for the While keyword concerning
placement within the loop apply to the Until keyword.
Using VBScript to Extend Your Web
Page
Now that you have learned some of the basics of VBScript, I want to walk you through
two examples of using VBScript to extend and enhance the functionality of your web
page. These examples are included on the CD-ROM with this book.
Validating User Input
The first example uses VBScript code to validate an input field for a form. The
script validates that the user enters a numeric value between 1 and 10. The application
displays a different message box based on a correct or incorrect entry. Listing 7.12
shows the web page and the VBScript code for this example.
Listing 7.12. Validating user input.
<HTML>
<HEAD>
<META NAME="GENERATOR" Content="Microsoft Developer Studio">
<META HTTP-EQUIV="Content-Type" content="text/html; charset=ISO-8859-1">
<TITLE>Validating User Input</TITLE>
<SCRIPT LANGUAGE="VBScript">
<!--
Sub Verify_OnClick
Dim MyForm
Set MyForm = Document.ValidForm
If IsNumeric(MyForm.txtEntry.Value) Then
If MyForm.txtEntry.Value < 1 Or MyForm.txtEntry.Value > 10 Then
MsgBox "Please enter a number between 1 and 10."
Else
MsgBox "Your entry was correct."
End If
Else
MsgBox "Please enter a numeric value."
End If
End Sub
-->
</SCRIPT>
</HEAD>
<BODY>
<H3> Validating the user input</H3><HR>
<FORM NAME="ValidForm" Action="">
Enter a value between 1 and 10:
<INPUT NAME="txtEntry" TYPE="TEXT" SIZE="2">
<INPUT NAME="Verify" TYPE="BUTTON" VALUE="Verify">
</FORM>
</BODY>
</HTML>
In Listing 7.12, I use an HTML form to provide the user interface. For the purposes
of this example, it isn't important that you understand how to implement an HTML
form. I will explain the use of HTML forms and controls on Day 12, "Using Basic
and Advanced HTML Form Controls." Figure 7.1 displays what happens when the
user types in an incorrect entry.
Figure 7.1.
An invalid entry.
Although this example is simple in nature, you should be able to get a feel for
how to integrate the power of VBScript into your HTML code. At the beginning of the
day, I explained that user input validation was an important capability of client-side
script. If you didn't use scripting code on the client, you would have to pass the
entry and validate the information with a program on the server. If the input is
wrong, you have to send a message back down to the client informing the user to enter
the data again. This process could be repeated several times before the user enters
a correct value for the field.
In Listing 7.12, you see the value of having the script resident within the web
page on the client. Once the user enters a value and presses the Verify button, the
input is instantly validated on the client machine. For the purposes of this example,
a message box is displayed informing the user whether the entry was valid or not.
Figure 7.2 shows the results of typing a correct value and pressing the Verify button.
Figure 7.2.
A valid entry.
In the context of a more robust application, you might pass a valid entry on to
the server for further processing.
Integrating VBScript with Controls
This next example illustrates how you can use client-side script to act as the
"glue" between multiple objects and controls within your web page. In the
example, I use an HTML Layout control to build the interface. You will learn how
to construct an application with ActiveX controls and the Layout control on Day 13,
"Interacting with Objects and ActiveX Controls," and on Day 15, "Integrating
Objects into Your Applications." For the purposes of the example, focus on the
way that VBScript interacts with the controls.
This application is a simple payment window that enables the user to enter the
payment type for an order. If the user chooses Cash or Check, the Credit Card and
Credit Card Number fields are disabled. Figure 7.3 demonstrates an example of selecting
the Cash option button.
Figure 7.3.
Selecting to pay by cash.
When the user clicks the Credit Card option button, the credit card information
fields are enabled, allowing the user to enter the credit card type and number. The
Submit button is enabled after the user enters information for both of these fields.
Figure 7.4 illustrates how the page looks when the user selects to pay by credit
card and enters the credit card information.
Listing 7.13 reveals the code that helps to integrate the activities of the different
controls on this page.
Figure 7.4.
Entering credit card information.
Listing 7.13. Selecting a payment
type.
Sub Layout2_OnLoad()
cmdSubmit.Enabled = False
End Sub
Sub optCash_OnClick()
call DisableCard()
End Sub
Sub optCheck_OnClick()
call DisableCard()
End Sub
Sub DisableCard()
txtCreditCard.Enabled = False
txtCardNumber.Enabled = False
lblCreditCard.Enabled = False
lblCardNumber.Enabled = False
End Sub
Sub optCredit_OnClick()
txtCreditCard.Enabled = True
txtCardNumber.Enabled = True
lblCreditCard.Enabled = True
lblCardNumber.Enabled = True
Call CheckFields()
End Sub
Sub txtCreditCard_Change()
Call CheckFields()
End Sub
Sub txtCardNumber_Change()
Call CheckFields()
End Sub
Sub CheckFields()
If txtCreditCard.Text = "" Or txtCardNumber.Text = "" Then
cmdSubmit.Enabled = False
Else
cmdSubmit.Enabled = True
End If
End Sub
You can sample this application on the CD-ROM with this book. The code for an
HTML Layout control is encompassed within the .alx file. You need to open this file
and use the Script Wizard to view the script for the different control events and
actions. You can see from the listing that user and system events trigger actions
that you can process within your script. These tasks should not be passed to the
server. For example, the credit card information fields are disabled when the user
selects to pay by cash or check. This user interface function is ideal for the client
to process.
Summary
You have ended the week on a very informative note. Hopefully, some of this information
served as a refresher for you. If you have used Visual Basic before, you should notice
the glaring similarities it has with VBScript. These similarities are only natural,
because VBScript is a subset of the Visual Basic language. This lesson attempted
to focus on the advantages of using a language like VBScript to enhance the functionality
of your web page from a client perspective. As you enter the second week, you will
discover the power that awaits on the server side of the equation.
Today you received an overview of how to use scripting code on the client, discovering
both the power and some of the drawbacks of client-side script. Next, you learned
about VBScript and JavaScript--two of the most widely used and popular scripting
languages today. The lesson presented a brief introduction and definition for each
of these languages. For further information on these languages, refer to Appendix
D, "VBScript Language Reference," and Appendix E, "JavaScript Language
Reference."
The latter part of the lesson provided you with some of the basics of VBScript.
This part of the lesson taught you some of the more robust features and capabilities
of the VBScript language. Finally, you saw some specific examples of VBScript and
web page interaction. You can inspect these examples further by accessing them from
the CD-ROM included with this book.
Q&A
- Q Can I use JavaScript and VBScript within my web page?
AWhile Visual InterDev supports the both JavaScript and VBScript, you can only
use one scripting language per page.
Q What is the difference between VBScript, VBA, and Visual Basic
AVisual Basic is the parent language for both VBA and VBScript. Visual Basic
provides both a robust language and development environment for client-server development.
VBA and VBScript are subsets of Visual Basic. VBA stands for Visual Basic for Applications
and is geared toward the power user. VBA is the programming language for the Microsoft
Office suite of applications. VBScript is yet another derivative that is geared specifically
for HTML web pages. VBScript can be used both on the client and the server side of
a Web-based application. Refer to the Visual InterDev online help for a comprehensive
list of VBA features that aren't included in VBScript.
Q What is a variant
AA variant serves as the lone data type for all variables in VBScript. The variant
can handle multiple data types, including numbers, text, dates, and objects. VBScript
categorizes the data that is stored within a variant through the use of subtypes.
These subtypes help VBScript to classify and perform operations on a variant.
Workshop
Using the code examples provided in today's lesson as well as the samples on the
CD-ROM, practice using some of the VBScript principles that you used today. Specifically,
you should become familiar with creating sub procedures and functions for your code.
Also, develop a script that takes advantage of the program control structures. After
developing this code, analyze the results to determine if the code acts the way you
think it should. Be prepared to answer the questions of why or why not.
You also should make a list of additional uses of client-side script, besides
the ones mentioned in this chapter. This list will help you better apply the concepts
that you learned today when you begin putting all of the pieces together to build
your application.
Quiz
- 1. What is the difference between Java and JavaScript?
2. What is the difference between a function and a sub procedure?
3. What is the difference between "Null" and "Empty"?
4. What does the ByVal statement do?
5. Given the following code segment, how many times will the code within the
loop execute before the loop terminates?
Sub cmdCalculate_OnClick()
Dim A, B, C
A = 10
B = 20
Do While A > B
C = A - B
A = A - B
Loop
End Sub
Quiz Answers
- 1. Java is a programming language designed to create applications and
applets, or "mini" applications. Java is a compiled language. JavaScript,
on the other hand, is an interpreted language that resides within HTML on a web page.
JavaScript is designed to provide scripting functionality to your web pages.
2 A sub procedure is a group of related code statements that work together to
complete a task. A function is different from a sub procedure in its ability to actually
return a value back to the program that called the function. A sub procedure cannot
return a value back to the calling program. A sub procedure and a function are two
types of procedures.
3 "Null" indicates that the variable has been intentionally set to
equal nothing. "Empty" represents a variable whose contents have not been
assigned a value.
4 The ByVal statement enables you to pass a variable by value to a procedure.
Passing a variable by value makes a copy of the variable for the procedure to access
and modify within the scope of that procedure. Any changes made to the variable within
the procedure aren't reflected back to the calling program.
5.The answer is zero. The Do...While loop executes a block of code as
long as a condition is true. The Do...While loop checks the condition first
before executing the code. If the condition is false, as in this case, the code within
the loop won't be executed.
|